12.5.3.1 code the movement of graphic objects;
Python. PyGame. Loading and animation pictures
Loading pictures
External pictures can be used as objects/surfaces.
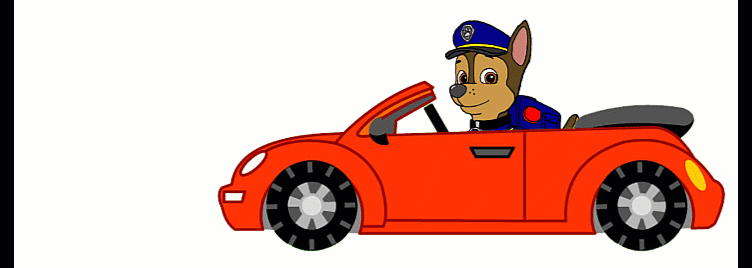
How to load the picture
Create an object (surface) and load a picture to this object.
surface_name = pygame.image.load('file_name’)
Example,
car = pygame.image.load('car.png’)
File .py and .png must be in the same folder.
We can use the full path. For example, "C:\\Python\\Projects\\ picture.png".
How to determine the size of the surface
w, h = surface.get_size() # w – width of surface, h – height of surface
or
w = surface.get_size()[0] # w – width of surface
h = surface.get_size()[1] # h – height of surface
or
# get_rect(object) - allows to get parameters of rectangle of surface
w = surface.get_rect()[2] # w – width of surface
h = surface.get_rect()[3] # h – height of surface
or
w = surface.get_width() # w – width of surface
h = surface.get_height() # h – height of surface
Transform module
pygame.transform.rotate(img, angle)
# rotate() - object rotation
# img - surface of object
# angle - counterclockwise rotation angle (in degrees)
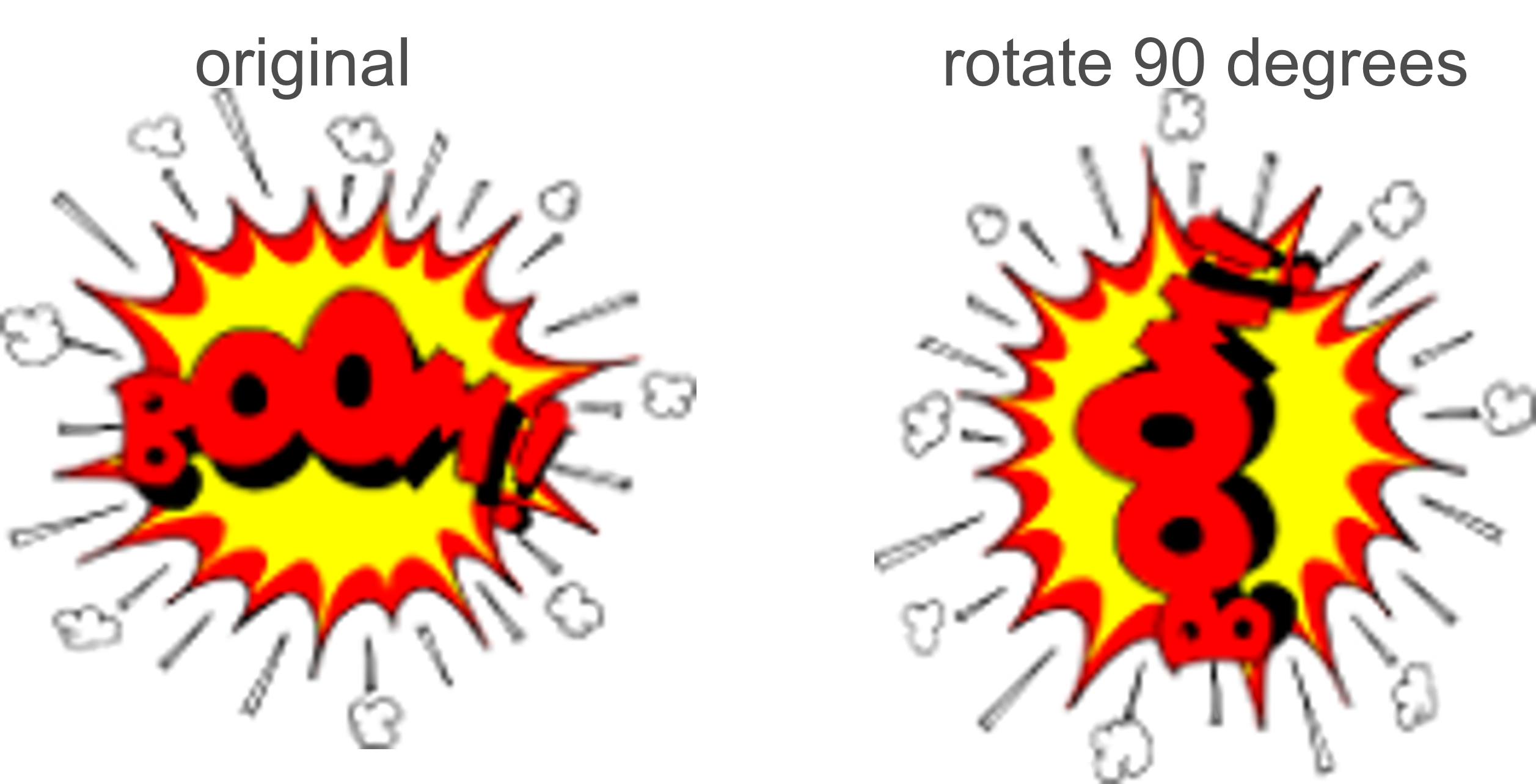
new_img = pygame.transform.flip(img, flip_x, flip_y)
# flip() - flip the surface
# img - surface of object
# flip_x - flip horizontally
# flip_y - flip vertically
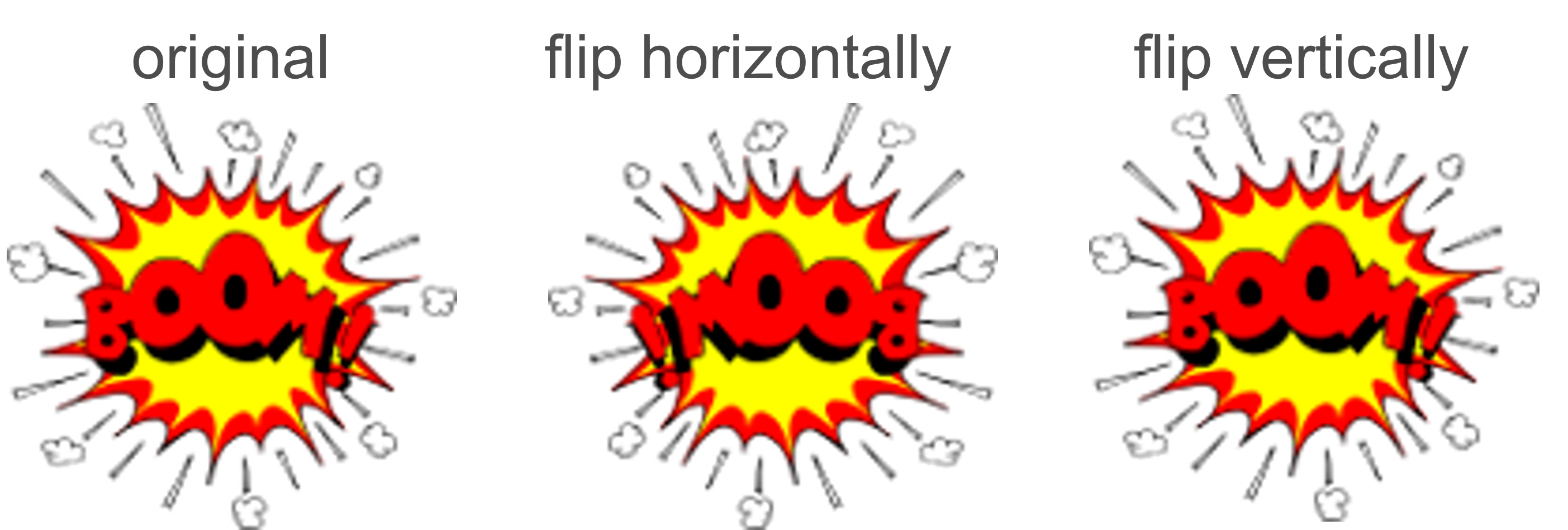
new_img = pygame.transform.scale(img, (width, height))
# scale() - change size of the surface
# width - size horizontally
# height - size vertically
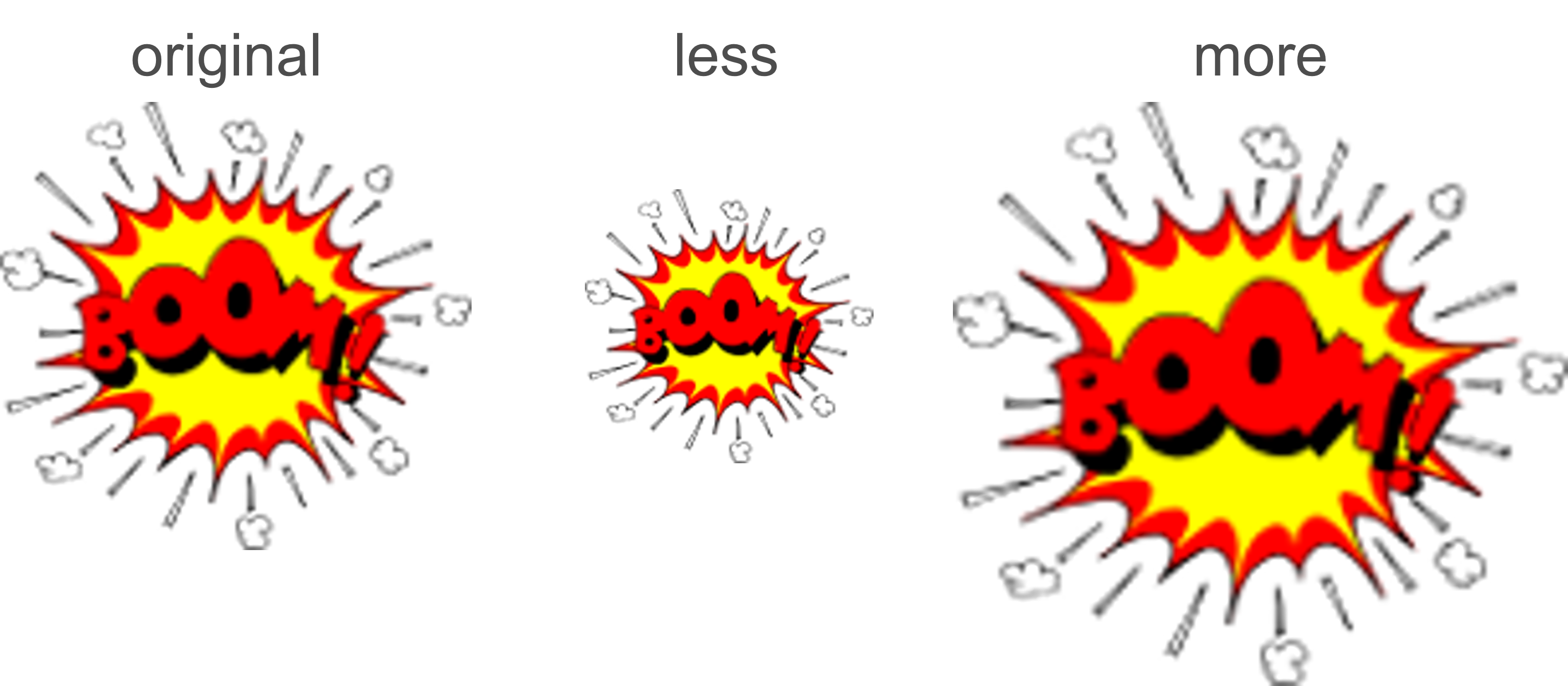
Save the surface as an image
We can save any surface as an image file.
pygame.image.save(surf, "filename")
# surf - surface which will be saved as a image file
# filename - name of file BMP, TGA, PNG or JPEG.
Creating a background for race
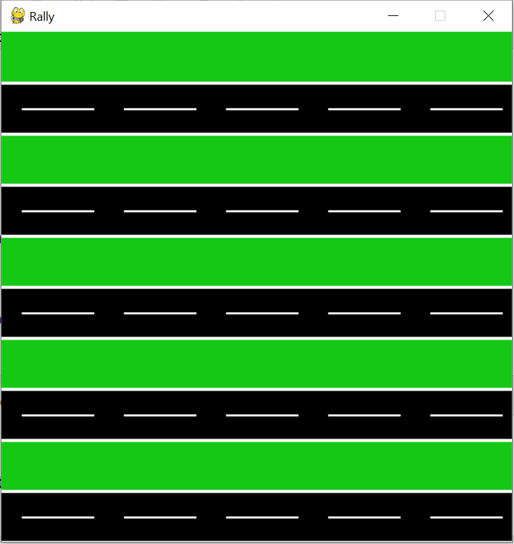
def road():
screen.fill((20, 200, 20))
rgb = (255, 255, 255)
for i in [50, 150, 250, 350, 450]:
pygame.draw.rect(screen, (0, 0, 0), (0, i, 500, 50), 0)
pygame.draw.line(screen, rgb, (0, i), (500, i), 3)
pygame.draw.line(screen, rgb, (0, i + 50), (500, i + 50), 3)
for j in [50, 150, 250, 350, 450]:
pygame.draw.line(screen, rgb, (j - 30, i + 25), (j + 40, i + 25),2)
Add car movement
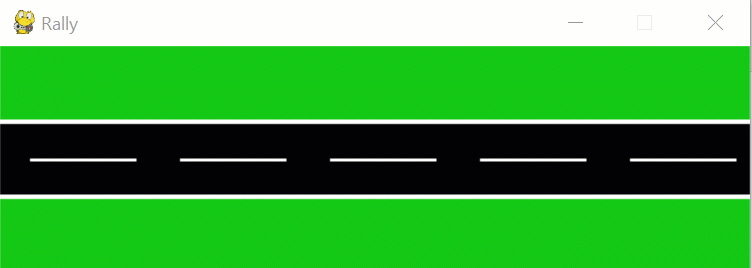
clock = pygame.time.Clock()
fps = 50
v = 200
car = pygame.image.load('car2.png')
w, h = car.get_size()
x = 20
k = 1
y = 60
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
road() # call a function
screen.blit(car, (x, y)) # output car on screen
if x > 500: # when the car went beyond the right border of the window
k = -1 # left direction
y = 40
car = pygame.image.load('car.png’)
if x < 1 - w: # when the car went beyond the left border of the window
k = 1 # left direction
y = 60
car = pygame.image.load('car2.png')
x += v / fps * k
clock.tick(fps)
pygame.display.flip()
pygame.quit()
Download pictures (click the right button --> Save picture as...)

Questions:
Exercises:
Tasks:
Tasks on Stepik.org course "Python Programming for NIS"
|