12.5.2.3 manage the surfaces programmatically.
Python. PyGame.
Surfaces
A surface is a layer of an object or space that can be managed separately from other objects.
Creating new surface
new_surface = pygame.Surface((width, height), pygame.SRCALPHA)
Surface - new surface
width - the width of the new surface
height - the height of the new surface
pygame.SRCALPHA - use transparency surface
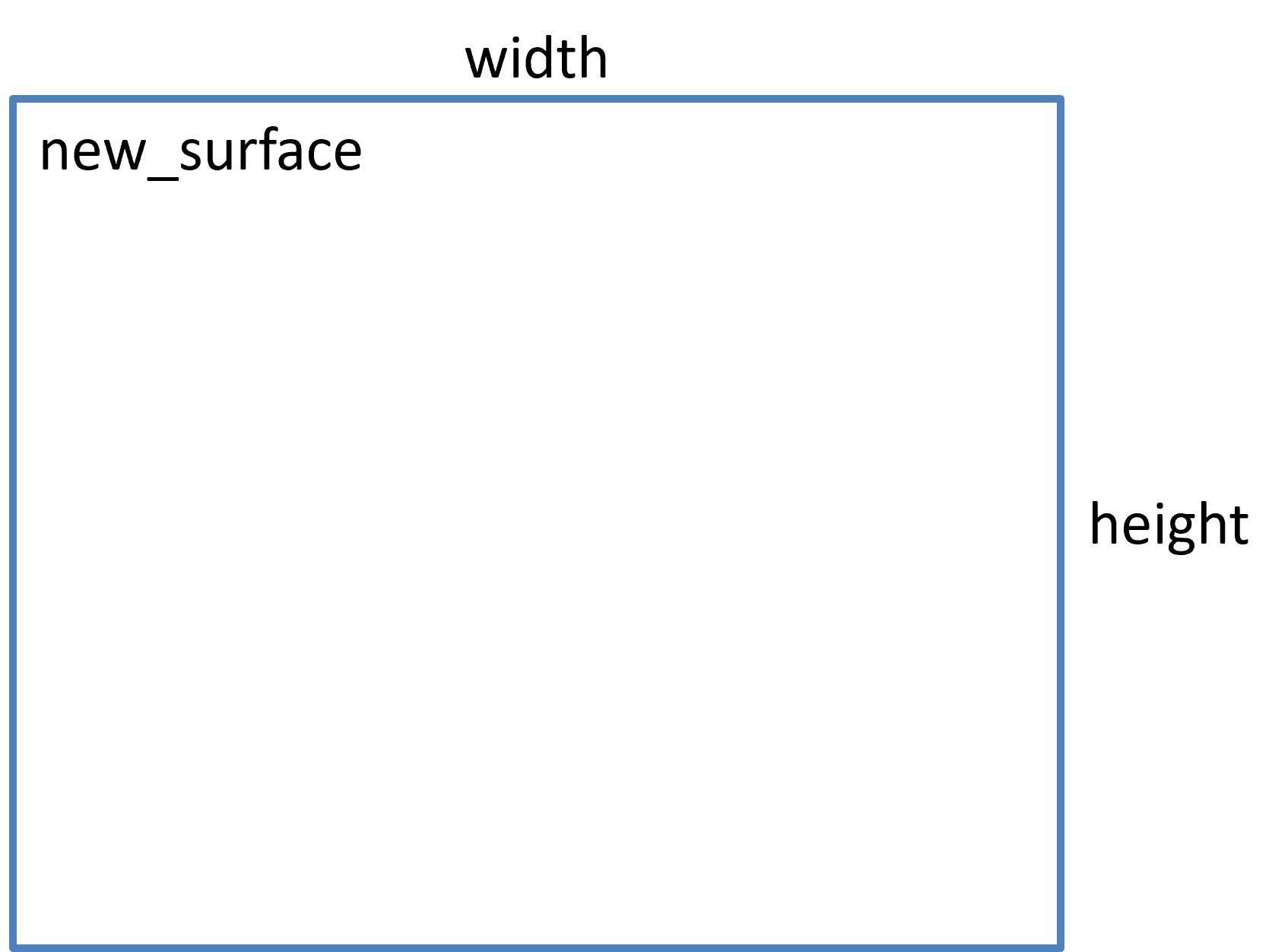
Output surface on display
screen.blit(new_surface, (x, y), rect)
screen - the main window or another surface
blit - method
bool - if True draw a line between the first and last points in the sequence of the points
rect - An optional area rectangle (x, y, width, height) can be passed as well. This represents a smaller portion of the source Surface to draw.
Output the whole surface:
new_surface = pygame.Surface((400, 400), pygame.SRCALPHA)
...
pygame.draw.circle(new_surface, (255, 0, 0), (50, 50), 50, 0)
screen.blit(new_surface, (50, 50))
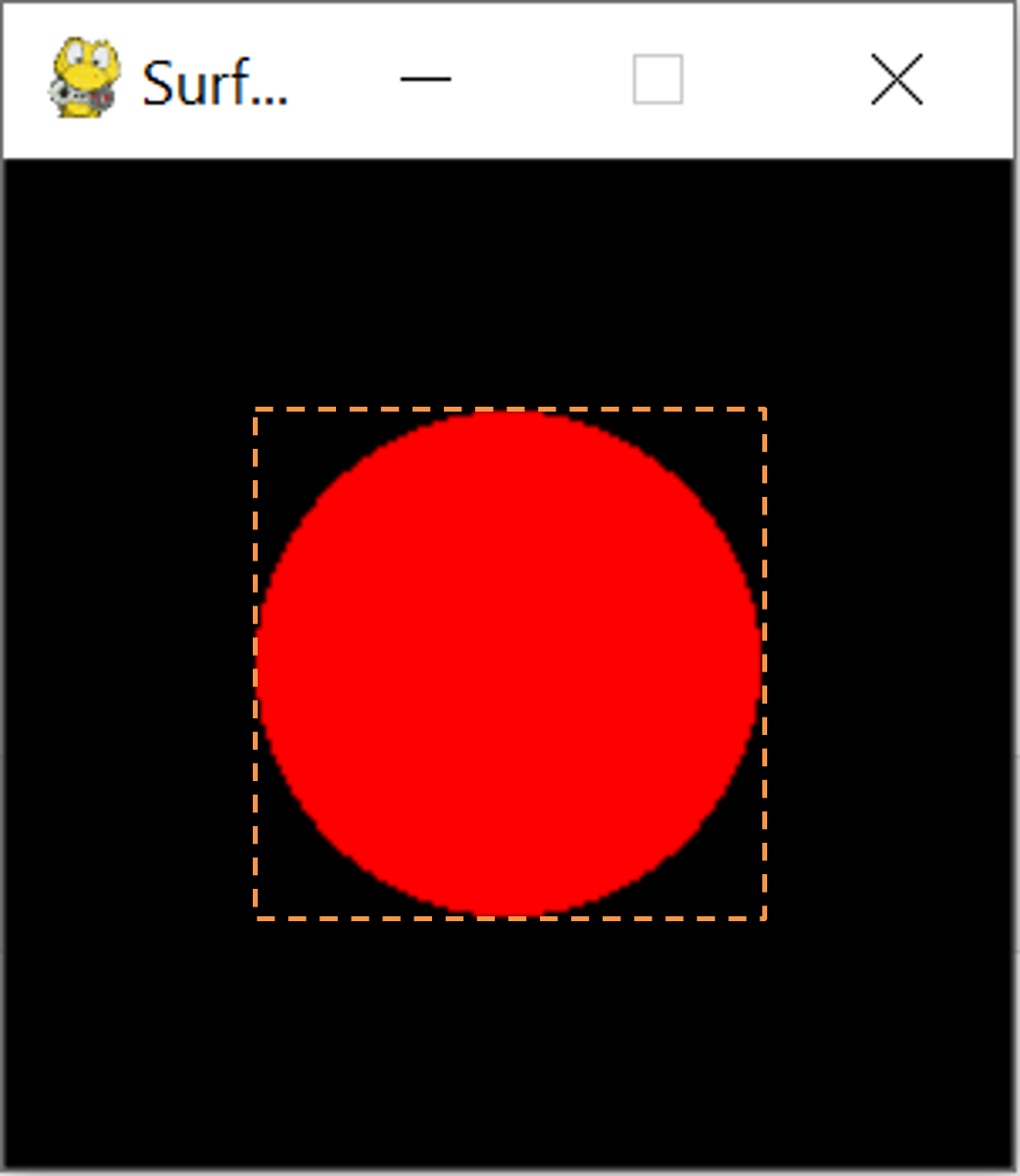
Output a part of the surface:
new_surface = pygame.Surface((400, 400), pygame.SRCALPHA)
...
pygame.draw.circle(p, (255, 0, 0), (50, 50), 50, 0)
screen.blit(new_surface, (50, 50), (0, 0, 50, 50)) 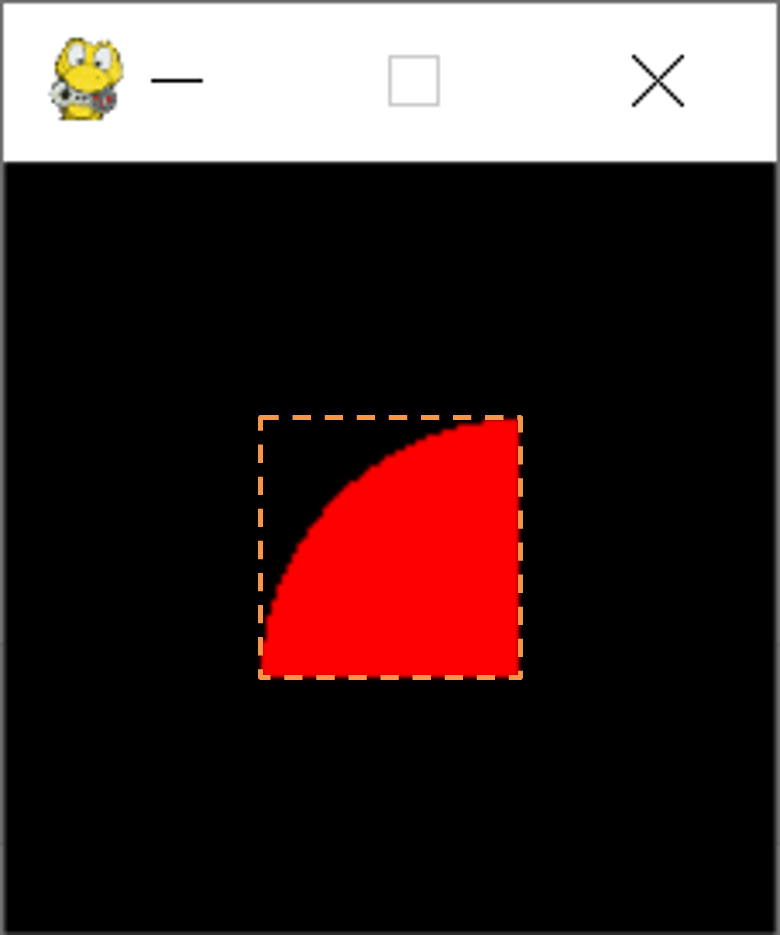
Output two surfaces on-screen game:
new_surface1 = pygame.Surface((400, 400), pygame.SRCALPHA)
new_surface2 = pygame.Surface((400, 400), pygame.SRCALPHA)
...
pygame.draw.circle(new_surface1, (255, 0, 0), (50, 50), 50, 0) # red circle
pygame.draw.circle(new_surface2, (255, 255, 0), (50, 50), 50, 0) # yellow circle
screen.blit(new_surface1, (50, 50)) # surface with red circle
screen.blit(new_surface2, (30, 30)) # surface with yellow circle When outputting multiple surfaces, the last output surface will be on the top layer.
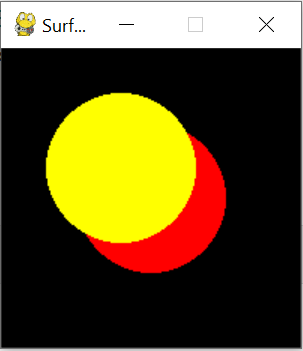
Output surface on another surface
new_surface1 = pygame.Surface((400, 400), pygame.SRCALPHA)
new_surface2 = pygame.Surface((400, 400), pygame.SRCALPHA)
...
pygame.draw.circle(new_surface1, (255, 0, 0), (50, 50), 50, 0) # red circle
pygame.draw.circle(new_surface2, (255, 255, 0), (50, 50), 50, 0) # yellow circle
screen.blit(new_surface1, (50, 50)) # surface with red circle
new_surface1.blit(new_surface2, (25, 25)) # surface with yellow circle When we draw the second surface on top of the first, the contents of the first surface will be in front.
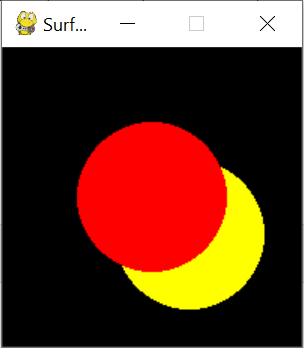
Example of the program
import pygame
pygame.init()
size = width, height = 600, 500
screen = pygame.display.set_mode(size)
pygame.display.set_caption('Surfaces')
new_surface1 = pygame.Surface((400, 400), pygame.SRCALPHA)
new_surface2 = pygame.Surface((400, 400), pygame.SRCALPHA)
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.draw.circle(new_surface1, (255, 0, 0), (50, 50), 50, 0)
pygame.draw.circle(new_surface2, (255, 255, 0), (50, 50), 50, 0)
screen.blit(new_surface1, (50, 50))
screen.blit(new_surface2, (25, 25))
pygame.display.update()
pygame.quit()
Questions:
1. Explain what is surface.
2. How to create a new surface.
3. What method is used to output one surface on another
Exercises:
Tasks:
Tasks on Stepik.org course "Python Programming for NIS" |