12.5.3.1 code the movement of graphic objects;
12.5.3.8 analyse the result of the program execution;
12.5.3.2 control the characters with module Time.
Python. PyGame.
Time and animation
Animation
The main idea of animation in programming is moving an object to a new place during the next iteration.
Consider the movement of the circle on the screen from right to left.
Before the game loop starts, we need to set the initial coordinates of the circle. For example:
x = 20
y = 100 To display an object in a new place, it is necessary to fill the screen with the background color every time before drawing.
screen.fill((0, 0, 0)) # fill the screen with black In the loop to draw the circle, we must use the variables (x, y) as the variable coordinates and at least one coordinate must change its value. For example:
pygame.draw.circle(screen, (255, 0, 0), (x, y), 20, 0)
x += 1 # movement to the right 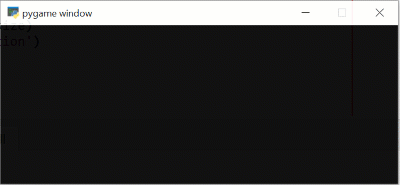
The animation is performed, but not at all as we would like. Time setting required.
Time
The easiest way is to set a method delay() from module time before the screen refreshes.
pygame.time.delay(n) # n - number of milliseconds
pygame.display.update() 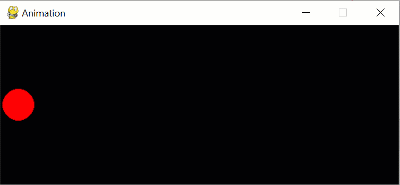
!!! The best way to use the Clock class of the time module to improve image quality.
clock = pygame.time.Clock() # create a new clock object
fps = 50 # frames per second (max 60)
v = 20 # speed: pixels per second
...
x += v / fps # new x-position
clock.tick(fps) # delay by 1/fps seconds 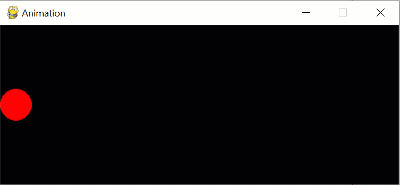
Example:import pygame
pygame.init()
size = width, height = 500, 200
screen = pygame.display.set_mode(size)
pygame.display.set_caption('Animation')
clock = pygame.time.Clock()
fps = 50
v = 40
x = 20
y = 100
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0))
pygame.draw.circle(screen, (255, 0, 0), (x, y), 20, 0)
x += v / fps
clock.tick(fps)
pygame.display.flip()
pygame.quit()
To limit the movement, it is enough to add the condition. For example:
if x < 480:
x += v/fps # movement to the right
# or
if x >= 20:
x -= v/fps # movement to the left
Questions:
1. Explain how to organize animation in programming.
2. Why do we use class Clock()?
3. How to implement movement to the right? left? upward? downward?
Exercises:
Ex. 1 Determine the direction of coordinate change using compass directions.
Tasks:
Tasks on Stepik.org course "Python Programming for NIS"
Task 1. Create infinity movement
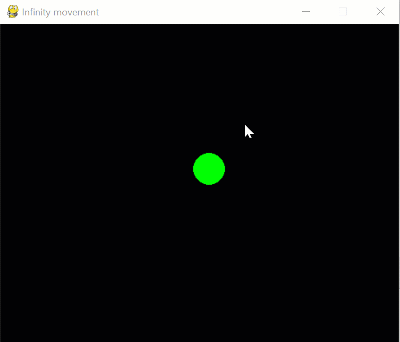 |