12.6.3.1 use commands of the Wave library to process sound files.
Wave library. Sound processing.
The wave module provides a convenient interface to the WAV audio format. It does not support compression/decompression, but it does support mono/stereo.
To open a *.wav file, use the function open()
wave.open(filename, mode=None)
filename - *.wav file which is in the *.py file directory.
mode = 'rb' - read-only mode.
mode = 'wb' - record-only mode.
import wave
source = wave.open("in.wav", mode="rb") # open file for reading
dest = wave.open("out.wav", mode="wb") # open file for writing Download in.wav
wave.close() - close *.wav file.
source.close()
dest.close()
to pass parameters from read file to write file use...
dest.setparams(source.getparams())
Frames of *.wav file
To get all the frames from an audio file, use the getnframes() function
frames_count = source.getnframes() Read frames in *.wav file
wave.readframes(wave.getnframes()) # read all frames from *.wav file.
Get information about wav-file
You can use:
(nchannels, sampwidth, framerate, nframes, comptype, compname) = source.getparams()
# getnchannels() - returns number of audio channels (1 for mono, 2 for stereo)
# getsampwidth() - returns sample width in bytes
# getframerate() - returns sampling frequency
# getnframes() - returns number of audio frames
Struct library
struct library — Interpret bytes as packed binary data
The pack() and unpack() methods. Packing and unpacking data.
struct.pack(format, v1, v2, ...)
Return a bytes object containing the values v1, v2, … packed according to the format string format. Allows you to pack audio fragment frames into a file.
struct.unpack(format, buffer)
Unpack from the buffer according to string format. Allows you to extract audio fragment frames to a file. The result is a tuple even if it contains exactly one item.import wave
import struct
source = wave.open("in.wav", mode="rb")
dest = wave.open("out.wav", mode="wb")
# copy parameters from source to dest
dest.setparams(source.getparams())
# find the number of frames
frames_count = source.getnframes()
# unpack all frames of in.wav to the list data
data = struct.unpack("<" + str(frames_count) + "h", source.readframes(frames_count))
# the main line of the program - reverse the list
newdata = data[::-1]
# pack all frames of data to the list newframes
newframes = struct.pack("<" + str(len(newdata)) + "h", *newdata)
# write content to the converted file out.wav
dest.writeframes(newframes)
source.close()
dest.close() Attention!!! In this tutorial, we will be working with *.wav audio files that are encoded unsigned 8-bit PCM in Audacity.
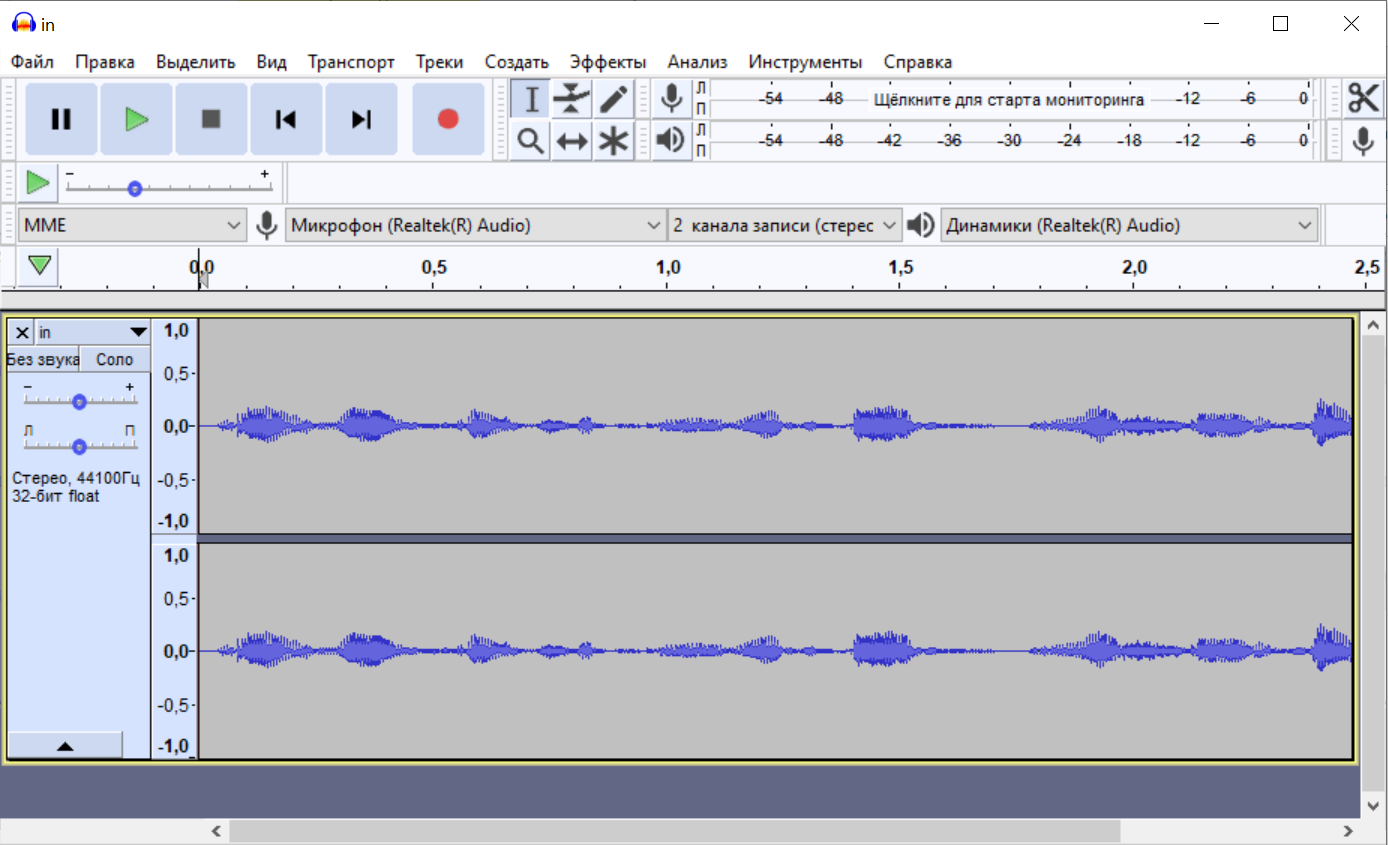
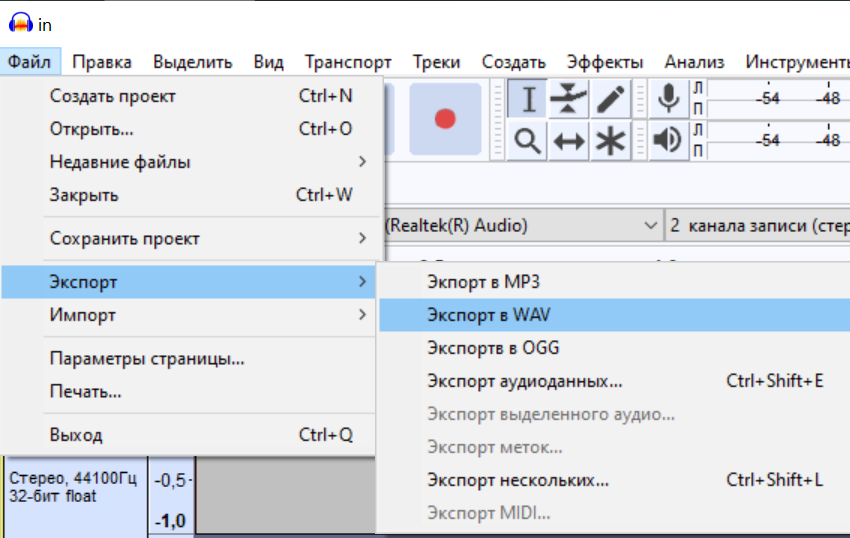
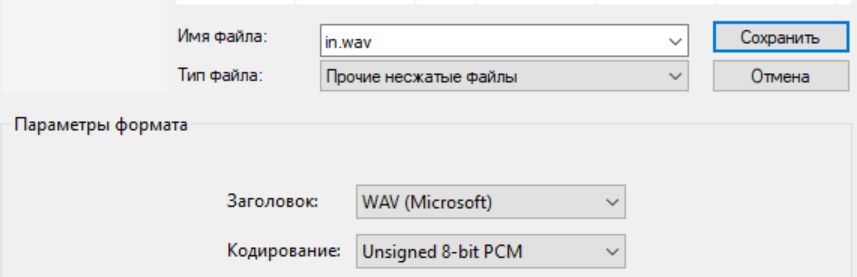
Questions:
Exercises:
Tasks:
Tasks on Stepik.org course "Python Programming for NIS"
-
Task "Copy *.wav file" Download in.wav.
Open in program file in.wav and create copy to out.wav.
-
Task "Count frames"
Output count frames of file in.wav.
-
Task "Half sound"
Output the first half part of file in.wav.
-
Task "Reverse sound"
Output to the out.wav reverse of file in.wav.
-
Task "Speed up twice"
Write a program to speed up a file in.wav twice.
-
Task "Slow down twice"
Write a program to slow down the sound twice of file in.wav.
|