12.5.2.1 output graphic primitives to the application window.
Python. PyGame.
Draw module
Draw Line
pygame.draw.line(screen, color, (x0, y0), (x1, y1), width)
screen - surface
color - color
(x0, y0) - the start of the line
(x1, y1) - end of line
width - width of the line
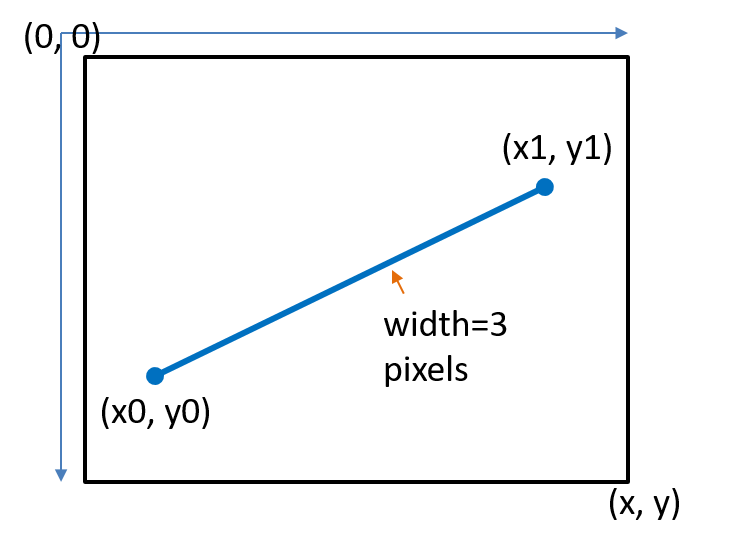
Draw Lines
pygame.draw.lines(screen, color, bool, [(x0, y0), (x1, y1), (x2, x2), ..., (xn, yn)], width)
screen - surface
color - color
bool - if True draw a line between the first and last points in the sequence of the points
[points] - points connected in series
width - width of the line
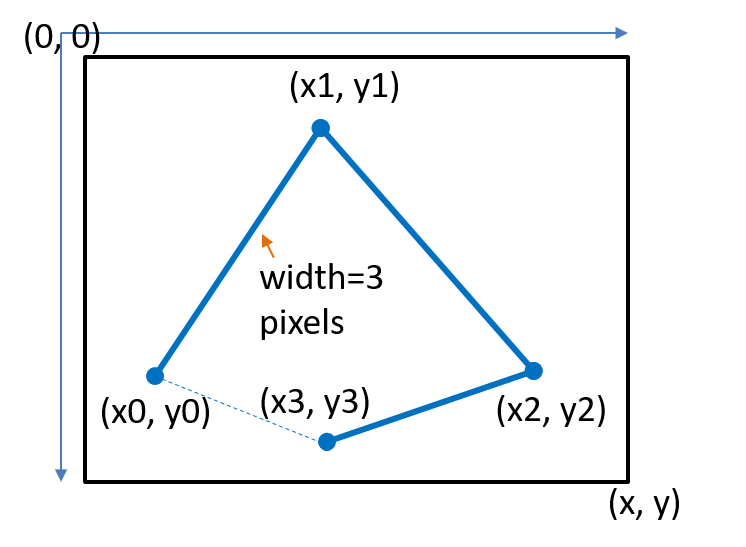
Draw Polygon
pygame.draw.polygon(surface, color, [points], width=0)
screen - surface
color - color
[points] - points connected in series
width - width of the line
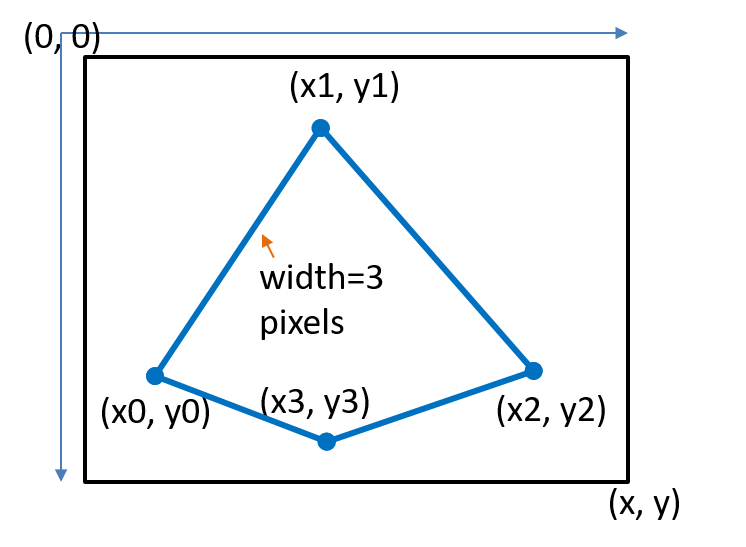
Draw Rectangle
pygame.draw.rect(screen, color, (x0, y0, width, height), border) screen - surface
color - fill color
(x0, y0, width, height) - coordinates of the upper left corner of the rectangle, the width of the rectangle, the height of the rectangle
border - the width of the border of the rectangle, with a value of 0, a filled rectangle is drawn
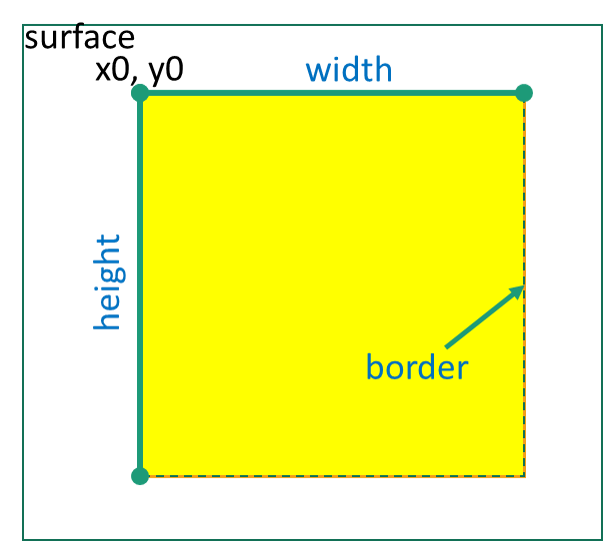
Draw Circle
pygame.draw.circle(screen, color, (x, y), R, border) screen – surface (поверхность)
color – fill color
(x, y) – координаты центра окружности
R – радиус окружности
border - the width of the border of the circle, with a value of 0, a filled circle is drawn
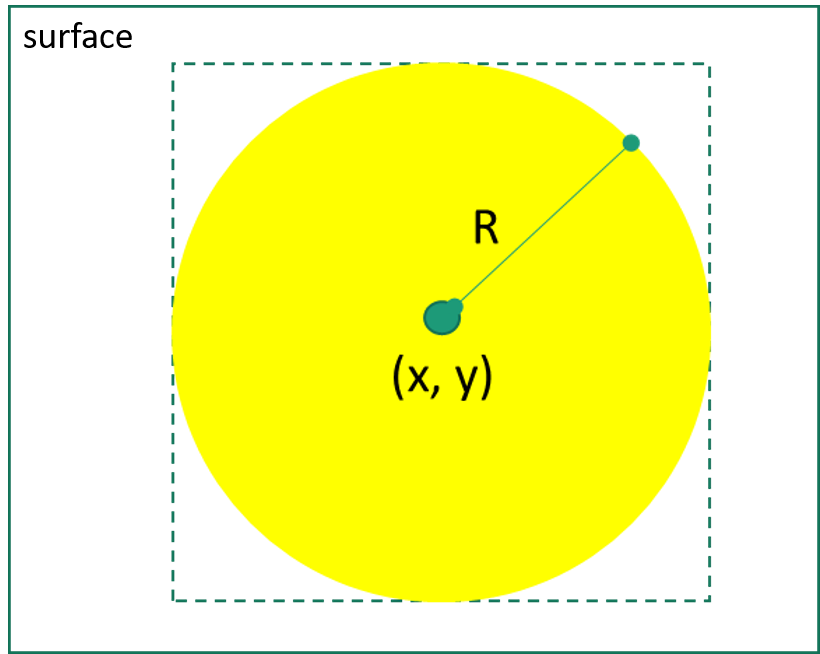
Draw Ellipse
pygame.draw.ellipse(screen, color, [x0, y0, width, height], width)
screen – surface (поверхность)
color – fill color
[x0, y0, width, height] – coordinates of the rectangle
border - the width of the border of the rectangle, with a value of 0, a filled rectangle is drawn
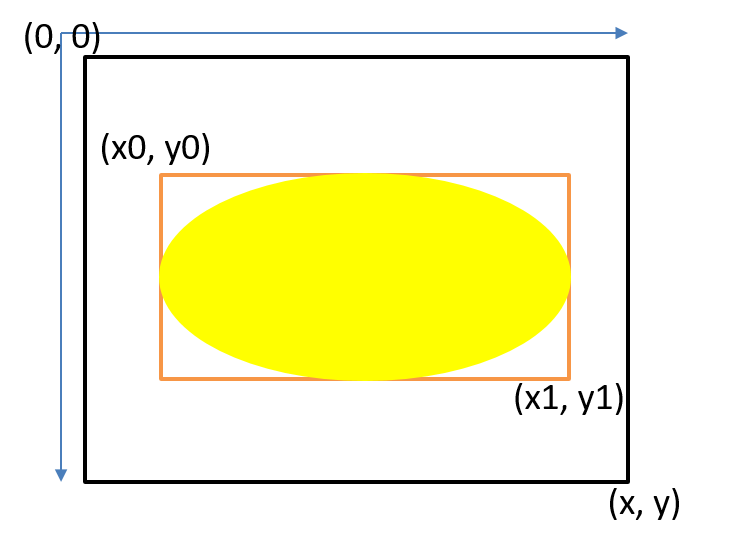
Draw Arc
pygame.draw.arc(screen, color, [x0, y0, width, height], start_angle, end_angle, width)
screen – surface
color – fill color
[x0, y0, width, height] – coordinates of the rectangle
start_angle (float) - start angle of the arc in radians
stop_angle (float) - stop angle of the arc in radians
width - width of the line
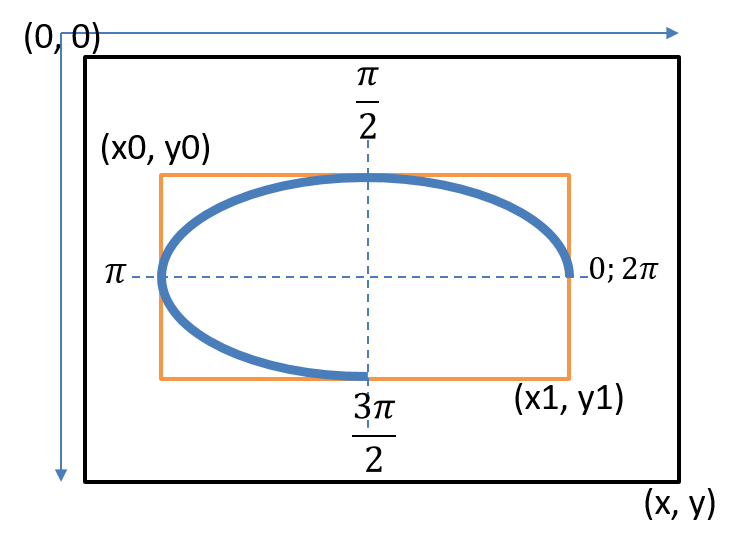
Example of programs
import pygame
pygame.init()
size = width, height = 400, 300
screen = pygame.display.set_mode(size)
pi=3.14
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.draw.arc(screen, (255, 255, 0), [100, 100, 200, 120],0, pi)
for i in range(5):
pygame.draw.arc(screen, (255, 255, 0), [100+40*i, 150, 40, 24],0, pi)
pygame.draw.line(screen, (255,255,0), (200, 150), (200, 200), 1)
pygame.draw.arc(screen, (255, 255, 0), [177, 189, 25, 25], pi, 2*pi)
pygame.display.flip()
pygame.quit()
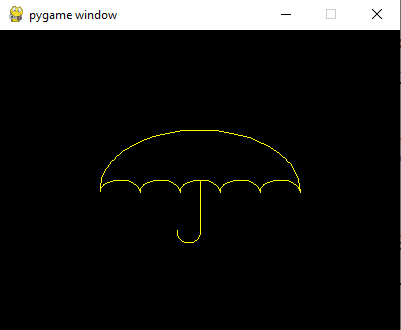
Questions:
Exercises:
Ex.1 Matching code and output if
size = width, height = 500, 500
screen = pygame.display.set_mode(size)
Tasks:
Tasks on Stepik.org course "Python Programming for NIS" |