12.5.3.3 control characters with the mouse.
Python. PyGame. Mouse events
Pygame has a mouse module. It allows you to get information about the device's mouse actions. We call them events.
An event is a user action. For example, the player moves the mouse, pressed the mouse button, etc.
At each stage of the main game loop, events are programmed to control the game.
Despite the fact that the loop is high-speed, there can be several events in one iteration. Therefore, a second inner loop appears in the program, which processes all the events that have occurred (breaks the sequence of events).
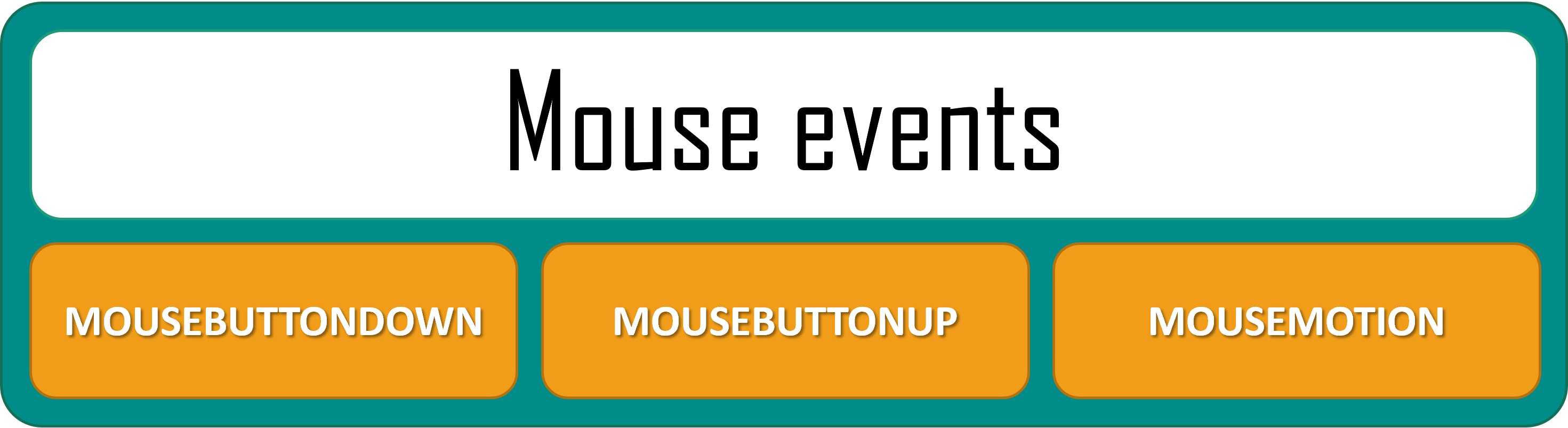
Pygame handles three types of mouse events:
- Press the button (the value of the type property of the event corresponds to the pygame.MOUSEBUTTONDOWN constant).
- Button release (pygame.MOUSEBUTTONUP).
- Mouse movement (pygame.MOUSEMOTION).
Mouse clicks
event.button=N - determines which mouse button is pressed.
N is...

For example,if event.button == 3: # if click right button
Cursor position
event.pos - allows you to get a tuple of two coordinates of the cursor location (x, y).
Example,
if event.type == pygame.MOUSEBUTTONDOWN:
x, y= event.pos # define the position of mouse pointer
pygame.draw.circle(screen, red, (x, y), 20) # draw circle with center of mouse pointer
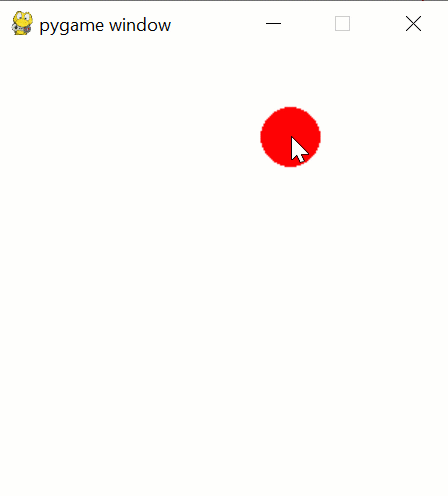
Task 1. Write a program in which the center of a circle with a diameter of 50 pixels moves after the cursor.
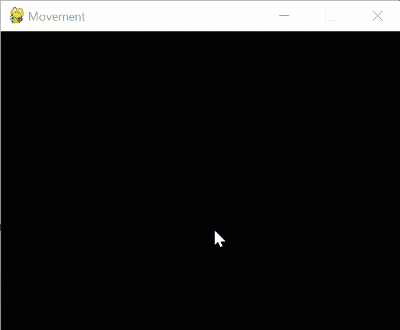
Solution:import pygame
size = width, height = 400, 300
screen = pygame.display.set_mode(size)
clock = pygame.time.Clock()
pygame.display.set_caption('Movement')
running = True
while running:
screen.fill((0, 0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEMOTION:
pygame.draw.circle(screen, (0, 0, 255), event.pos, 20)
clock.tick(50)
pygame.display.flip()
pygame.quit() In order for the circle not to disappear from the screen, you need to draw it in a loop, and get the coordinates when you move the mouse:while ...
...
if event.type == pygame.MOUSEMOTION:
pos = event.pos # get the coordinates
...
pygame.draw.circle(screen, (0, 0, 255), pos, 20) # draw circle
... Task 2. Display text according to the mouse button pressed.if event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1: # if pressed left button
print("You pressed left button")
if event.button == 3: # if pressed right button
print("You pressed right button")
Drag and drop
The algorithm for working with dragging an object:
- When you click the mouse button, turn on the movement mode
- When moving, change the position of the coordinates (x, y) and redraw the object
- When you release the mouse button, turn off the move mode
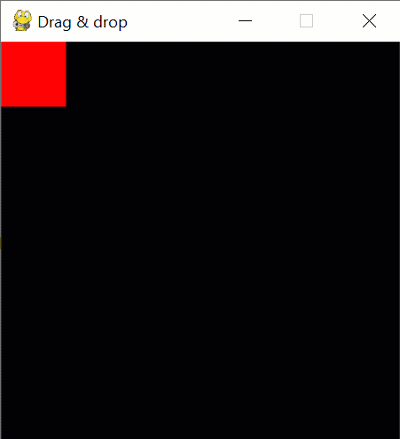
Solution:
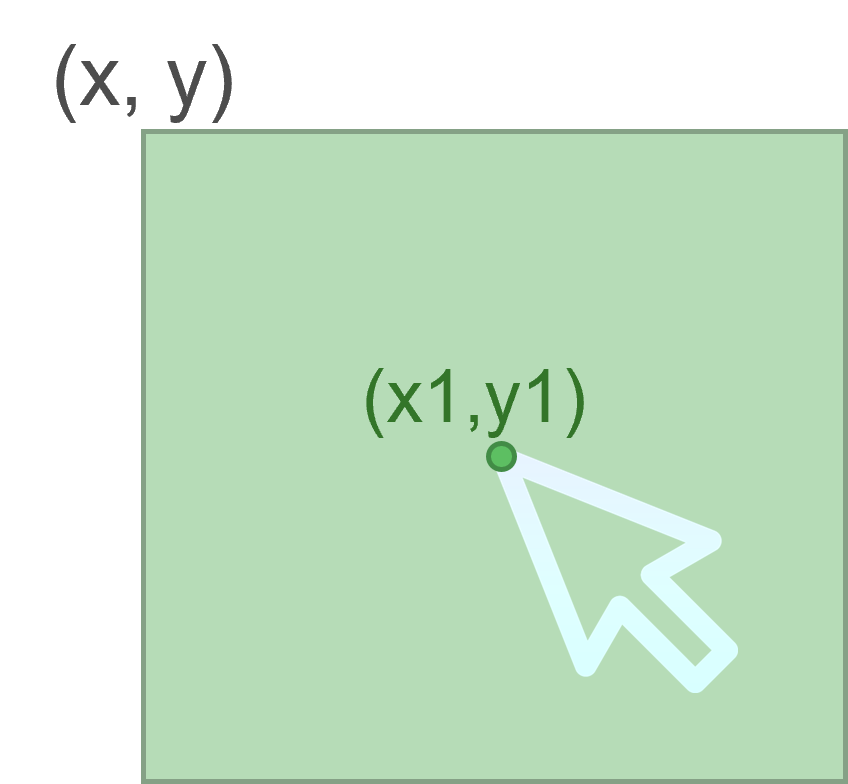 import pygame
pygame.display.set_caption('Drag & drop')
size = width, height = 300, 300
screen = pygame.display.set_mode(size)
clock = pygame.time.Clock()
x1, y1, x, y = 0, 0, 0, 0 # start location
moving = False
running = True
while running:
screen.fill((0, 0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEBUTTONDOWN:
# check if mouse pointer is in square
if x1 <= event.pos[0] <= x1 + 50 and y1 <= event.pos[1] <= y1 + 50:
moving = True
# determine the distance to the top left corner of the square
x, y = event.pos[0] - x1, event.pos[1] - y1
if event.type == pygame.MOUSEBUTTONUP:
moving = False
if event.type == pygame.MOUSEMOTION:
if moving:
# set the coordinates of the top left corner of the square
x1, y1 = event.pos[0] - x, event.pos[1] - y
screen.fill((0, 0, 0))
pygame.draw.rect(screen, (255, 0, 0), ((x1, y1), (50, 50)))
clock.tick(50)
pygame.display.flip()
pygame.quit()
Questions:
1) Name the mouse event when you can program it in PyGame.
2) Explain how to organize movement according to the mouse pointer.
3) Describe the algorithm implementing the action "drag & drop".
Exercises:
Tasks:
Tasks on Stepik.org course "Python Programming for NIS"
|